How to create Array.prototype.map polyfill in javascript ?
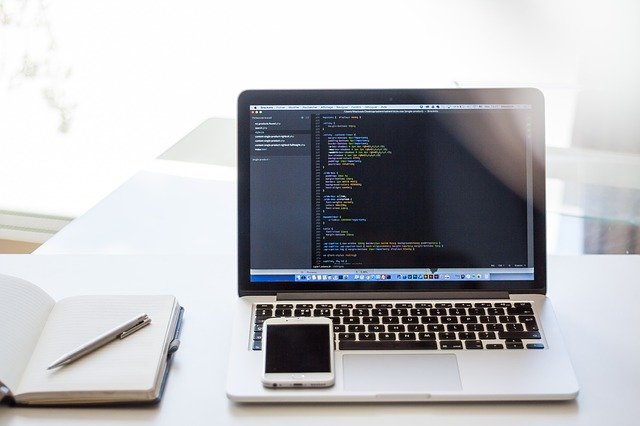
Array.prototype.map is used to loop over array & return new array. You can use this map to manipulate your array & get the changed array. If you don’t want new array in return then you shouldn’t use map, use forEach instead. If you want to learn prototypes first, please go here.
Today we will see how a developer should approach for creating any polyfill, map polyfill to be specific. This tutorial is for learning purpose only, if you are looking for ready made production ready polyfill of map, kindly visit mdn official site here.
Approach for creating map polyfill
Array.prototype.map = (callback=>{
var newArr = [];
for(let i=0; i ◀️ this.length; i++){
newArr.push(callback(this[i], i));
}
return newArr;
})
If you understood the code given above then you know the basics of javascript and you should move to another advanced tutorial for new learning. In the steps below we will keep referring the above polyfill.
Capture the INPUT
Before starting the coding for polyfill, first make sure you know about how your code will capture input the data used in map. So, input is very important in this case. For example:
const myArr = ["apple", "boy", "cat"]
myArr.map(data=>{
//data will be accessible here
})
//while creating polyfill, think how will you access myArr in custom map ?
“this” keyword will be help us in providing the input to our custom map polyfill. Just checkout the polyfill code on the top & see how I used this keyword. Example: this.length will give me myArr.length in my polyfill.
Logic
This step should be simpler for you if you have good knowledge of data structure and algorithms, so let’s not elaborate this for now. So, just have a look on the polyfill code, you will get the idea what we are doing there.
Return the output
In this step, make sure you return the proper data type. In our case we know that map return new array only. So, we will create new array & based on the logic we will just return this new array. This is what we did in our example above.
Let’s Conclude
While giving frontend developer interviews, the Interviewer is mostly interested in the javascript knowledge of the interviewee. Asking Polyfill related questions help the interviewer to judge the pure vanilla JS knowledge of the candidate. My two cent is to create 1 or 2 polyfills before giving the interview test, you can start from very basic which is given in this tutorial. Happy Interviewing 🙂