What is CORS ? How to resolve this issue from frontend ?
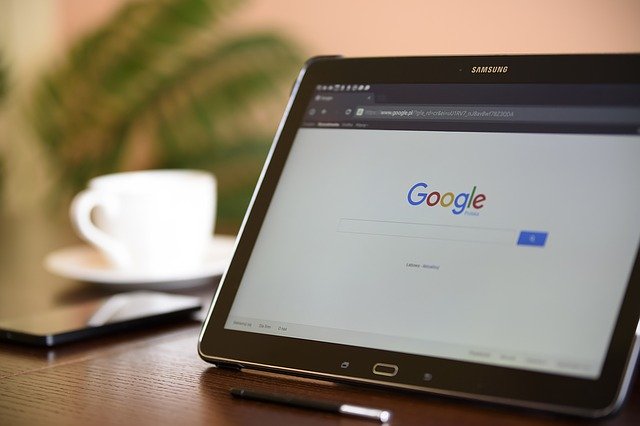
CORS stands for “Cross-origin resource sharing” which is the security policy set by the browsers to block different domain request unless the origin is whitelisted in other domain.
Resolve CORS
CORS issue can be resolved only from backend/server, so if you are thinking from frontend you can resolve then it’s not possible. Now let’s look at several cases of CORS error & their solutions.
Preflight Request (OPTIONS request)
This is important step if your frontend is different from backend server, all browsers first make OPTIONS request to server to check whether server allows the request from origin. Now how do browsers know whether server has allowed some origin ? response header is your answer.
Server needs to allow OPTIONS method & sends CORS header to tell browser that let the origin requests come to us. Let’s discuss different CORS header server should send in response.
Access-Control-Allow-Origin
Access to fetch at ‘domainname.com/’ from origin ‘https://wmnitin.dev’ has been blocked by CORS policy: No ‘Access-Control-Allow-Origin’ header is present on the requested resource. If an opaque response serves your needs, set the request’s mode to ‘no-cors’ to fetch the resource with CORS disabled.
Whenever a request goes from browser, it automatically sends origin request header to server, when browser finds that server is not allowing this origin then the browser blocks this request & doesn’t send any request to server.
To solve this CORS issue, server needs to add response header like this
access-control-allow-origin: *
or
access-control-allow-origin: domainname.com
Remember only * or single domain is allowed in this field otherwise browser will throw multiple origin error.
Access-Control-Allow-Headers
Error: XMLHttpRequest cannot load https://wmnitin.dev. Request header field content-type is not allowed by Access-Control-Allow-Headers in preflight response
This error comes because we need to allow some client headers from server side. Apart from sending allow-origin header, server should allow extra headers that is being sent in request header by client.
To solve this CORS issue, server needs to add response header like this:
access-control-allow-headers: 'AnyHeaderName, content-type'
Remember, wildcard (*) is not allowed in this header, so put all incoming headers manually to remove this CORS issue
Access-Control-Allow-Methods
This response header is as much important as other other CORS headers. You need to allow & tells the browser what http methods are allowed for the incoming request. Server can allow any methods, remember, wildcard is not allowed in the value of this response header.
To solve this CORS issue, server needs to add response header like this:
access-control-allow-methods: 'POST, GET, OPTIONS'
Temporary Resolve CORS from Front-end
If you want to fix this error without the help from backend/server then you need to tell the browser to disable this CORS policy for you. Remember this solution will only work for you until you are developing frontend, other users will still see CORS error.
To solve this CORS issue, you need to remove CORS policy browser like this:
You need to boot Chrome browser in unsafe mode using below command
"[PATH_TO_CHROME]\chrome.exe" --disable-web-security --disable-gpu --user-data-dir=~/chromeTemp.
This will disable CORS browser policy for you, as we said this is only temporary solution. This CORS issue should be resolved by server by adding response header
Access-Control-Allow-Origin: https://wmnitin.dev
Access-Control-Allow-Methods: POST, GET, OPTIONS
Access-Control-Allow-Headers: X-PINGOTHER, Content-Type, anyHeader